Command |
DRAW |
|
|
Description |
Draw or update a Line, Vector, Box, Circle or Graph of size X,Y. The entities can be an outline or filled.
The colour can be enhanced using alpha blending within the draw style.
Graphs of a different colour can be superimposed on top of each other.
Faster display updates occur if draw uses a solid background colour (ie no alpha blending).
DRAW accepts VARs, signed/unsigned integers
(U8, U16, U32, S8, S16, S32), floats (FLT) and pointers (PTR)
DRAW( PTR, VAR|INT|FLT|PTR, VAR|INT|FLT|PTR, Style );
Note PTR refers to the entity being pointed to by PTR and not
the PTR itself. Use LOAD( PTR > "Name" ); to set a pointer. |
|
|
Syntax/Parameters |
DRAW(Name,X,Y,Style)
DRAW(Name,X,Y,Style,PosX,PosY);
DRAW(name,length,angle,stVector); |
|
|
Style |
It is possible to specify transparency values with colours if the colour is entered as a 32-bit hex number the top 8 bits specify the alpha blending level.
col = \\aarrggbb; back = \\aarrggbb; where aa = alpha level.
For example, col = \\80FFFF00; gives 50% transparent yellow.
Support for opacity - v49.00.
STYLE(gstyle,DRAW) {
type=trace; //The shape to draw. type = B/Box; C/Circle; L/Line;, T/Trace; P/Pixel; y/yBar; x/xBar;
v/Vector;
maxX=100; // Not required except for dynamic rotation where the maximum width is declared
maxY=100; // Not required except for dynamic rotation where the maximum height is declared
col=green; //Specify the line border colour of the shape. Use hex, colour name + alpha
back=black; //Specify the fill colour of the shape. Use hex, colour name + alpha
opacity = n; // n = 0..100 where 0=transparent..100=opaque (default=100)
width=3; //Specify the line border width of the shape default = 1
rotate=0; // Specify the rotation of the shape with respect to the screen. 0,90,180,270
curRel=cc; //specify placement relative to cursor. CC Centre Centre , TC Top Centre etc.
xOrigin=50; //specify graph x origin wit respect to declared graph
yOrigin=50; //specify graph y origin wit respect to declared graph
xScale=200; //scale the value automatically to fit the graph
yScale=200; //scale the value automatically to fit the graph
xScroll=1; //define scroll direction and increment 1=left to right one pixel, 0=no
scroll
} |
|
|
Options |
Graphs - v47.00
A number of graph styles now exist as draw types:
type=p; type=pixel; // Pixel Scatter - places a point at x,y
type=t; type=trace; // Trace/Line - joins the dots between current point and previous point.
type=y; type=yBar; // Bar Y - draws vertical line from 0 to y and clears from y+1 to ymax
type=x; type=xBar; // Bar X - draws horizontal line from 0 to x and clears from x+1 to xmax
The origin on the graph can be changed
xOrigin=val; // (default=0)
yOrigin=val; // (default=0)
The scaling of pixels can be set:
xScale=val; // (default=100.0) [val can be float and is a percentage]
yScale=val; // (default=100.0) [val can be float and is a percentage]
Note to draw graph with 0,0 at top and n,n at bottom, use yScale=-100;
The graph can be made to scroll (currently right-to-left only supported)
xScroll=val; // where val=0 (default - no scroll); val=n (scroll left n pixels before each plot
Please refer to the ADC analogue input section for a graph application example.
Graph Displaying Problems v49.37
* Added bounds check to prevent drawing outside of graph
area.
* Fixed line wrapping within graph area.
DRAWing of Graphs - Trace Line Should
Not be Wrapping - v49.42
* Improved graph trace drawing.
* Solved problem with trace line wrapping when rendering a graph.
* Extended graphics area to encompass line width and modified page rendering to
deal with windowed pixels.
DRAWing of Ellipses and more DRAW parameter options
- v49.37
* Added ellipse drawing, specify type=ellipse or e in the
STYLE
* Added more DRAW command options
DRAW( name, x ); // Circle or Square modification: width=x; height=x;
DRAW( name, x, style ); // Circle or Square definition: width=x; height=x;
DRAW( name, x, y ); // All Shapes modification: width=x; height=y;
DRAW( name, x, y, style ); // All Shapes definition: width=x; height=y;
DRAW( name, x, y, s, a ); // Ellipse Arc modification: width=x; height=y;
startAngle=s; arcAngle=a;
DRAW( name, x, y, s, a, style ); // Ellipse Arc definition: width=x;
height=y; startAngle=s; arcAngle=a;
* Notes:
- 0 degrees is at 12'o'clock and positive angles are clockwise.
- The style for an arc must be defined to encompass the size of the shape if
drawn from 0 to 360 degrees.
- Further work required on the fill algorithm for ellipses.
* Added option for start angle and
arc angle to be in radians or degrees depending on SYSTEM.angles setting.
v49.48
Graphs to support alpha blending
and opacity - v49.42
* New features of alpha blending and opacity with graphs
* Support for graphs when the display is rotated 90, 180 and 270 degrees
Drawing Lines - v49.48
* New algorithm for line drawing
implemented.
* Lines are created with anti-aliased edges.
* Vertical and horizontal lines are a special case and are rapidly created (as
no anti-aliasing required).
* Line ends are now created perpendicular to the line rather than vertical or
horizontal.
Drawing Vectors (Lines with length and angle) - v49.48
* Added new line type - vector - which is defined with a length and an angle.
STYLE( stVector, DRAW ) { type = v or vector; ... }
DRAW( name, length, angle, stVector );
where
'length' is line length,
'angle' is angle clockwise from 12'o'clock position. 'angle' is in degrees or
radians depending on SYSTEM.angles setting.
Example tu800a.mnu
STYLE(stPage,PAGE){}
STYLE(stLine1,DRAW){type=vector;col=white;width=1;curRel=cc;}
STYLE(stLine2,DRAW){type=vector;col=white;width=2;curRel=cc;}
STYLE(stLine3,DRAW){type=vector;col=white;width=3;curRel=cc;}
STYLE(stLine4,DRAW){type=v;col=white;width=9;curRel=cc;}
STYLE(stLine5,DRAW){type=v;col=white;width=20;curRel=cc;}
STYLE(stLine6,DRAW){type=v;col=white;width=21;curRel=cc;}
STYLE(stCirc,DRAW){type=circle;width=1;col=red;curRel=cc;}
VAR(varAngle,0.0,FLT4);
PAGE(pgMain,stPage)
{
POSN(149,119); DRAW(drLine1,200,45,stLine1); DRAW(drCir1,150,stCirc);
DRAW(drPt1,7,stCirc);
POSN(399,119); DRAW(drLine2,200,45,stLine2); DRAW(drCir2,150,stCirc);
DRAW(drPt2,7,stCirc);
POSN(649,119); DRAW(drLine3,200,45,stLine3); DRAW(drCir3,150,stCirc);
DRAW(drPt3,7,stCirc);
POSN(149,359); DRAW(drLine4,200,45,stLine4); DRAW(drCir4,150,stCirc);
DRAW(drPt4,7,stCirc);
POSN(399,359); DRAW(drLine5,200,45,stLine5); DRAW(drCir5,150,stCirc);
DRAW(drPt5,7,stCirc);
POSN(649,359); DRAW(drLine6,200,45,stLine6); DRAW(drCir6,150,stCirc);
DRAW(drPt6,7,stCirc);
LOOP(lpMain,FOREVER)
{
DRAW(drLine1,150,varAngle);
DRAW(drLine2,150,varAngle);
DRAW(drLine3,150,varAngle);
DRAW(drLine4,150,varAngle);
DRAW(drLine5,150,varAngle);
DRAW(drLine6,150,varAngle);;
CALC(varAngle,varAngle,0.5,"+");
}
}
SHOW(pgMain);
New Line Draw CurRel options plus offset - v49.48
* Additional parameter can now be passed to DRAW() command for Line and
Vector drawing which allow for the line to be offset relative to the cursor:
DRAW( name, length, angle, offset, stVect );
DRAW( name, length, angle, offset );
DRAW( name, length, angle, offset, stVect, xPos, yPos );
DRAW( name, width, height, offset, stLine );
DRAW( name, width, height, offset );
DRAW( name, width, height, offset, stLine, xPos, yPos );
* The offset parameter is ideal for the pointer on gauges and clocks where the
point of rotation is a certain distance along the line
* New curRel options allow for better line placement.
SA, SB, SC // Start of line. SA = Edge A; SB = Edge B; SC = Centre
EA, EB, EC // End of line. EA = Edge A; EB = Edge B; EC = Centre
MA, MB, MC // Mid-point of line. MA = Edge A; MB = Edge B; MC = Centre
OA, OB, OC // Offset along line. OA = Edge A; OB = Edge Bl OC = Centre
* Example: Vector length = 100, angle = 125 deg, width = 5 (not to scale)
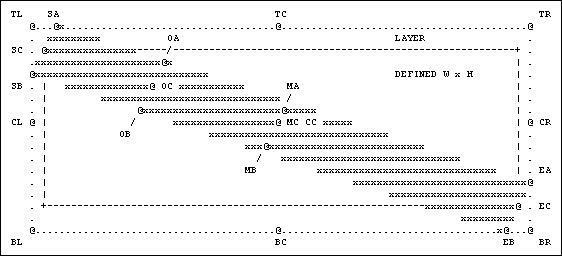
Draw() with structures - v49.48
* DRAW() command now accepts structure parameters for
width/height/angles/offset.
Anti-Aliased Line Drawing - v49.48
* New algorithm for line drawing implemented.
* Lines are created with anti-aliased edges.
* Vertical and horizontal lines are a special case and are rapidly created (as
no anti-aliasing required).
* Line ends are now created perpendicular to the line rather than vertical or
horizontal. |
|
|
Example |
Circle Example
DRAW(MyCircle, 32, 32, DrawCircle);
DRAW(MyCircle, 64, 64); //modified circle is double diameter.
DRAW(MyCircle,VarX,VarY); //modified
circle using variables. Should not exceed MaxX,maxY.
Box Example
DRAW(MyBox, 32, 32, DrawBox);
DRAW(MyBox, 64, 64); //modified circle is double diameter.
DRAW(MyBox,VarX,VarY); //modified
circle using variables. Should not exceed MaxX,maxY.
Line Example
DRAW(MyLine,10,10,lineStyle); //draws line 45 degrees top left to bottom right.
DRAW(MyLine2,10,-10,lineStyle); //draws line 45 degrees bottom left to top right.
Vector Example
DRAW(MyVec,150,30,VecStyle); //draws vector 150 length at 30 degree.
DRAW(MyVec2,100,320,VecStyle); //draws vector 100 length at 320 degree.
Graph Example
DRAW(MyGraph,100,100,GraphStyle); //draws a graph window of 100x100 pixels.
DRAW(MyGraph,20,30); //draws a pixel on the graph at 20,30 relative to the origin.
DRAW(MyGraph,varX,varY); //use variables to plot a pixel on the graph.
RESET(MyGraph); //clears the graph |
|
|
Update Information |
Version |
Title |
Date |
Details |
49.58 |
Line style update |
07 Sep 15 |
|
* Fixed problem when switching or changing the style of a horizontal or vertical line not updating.
|
|
49.52 |
Built-In Styles - Parameters Are Optional |
25 Feb 14 |
|
Modified "built-in" styles to make parameters optional. Simply miss out the parameter between the underscores.
TEXT(txt4,"",DST___128__CC); // Create Text with only maxLen and curRel changed from defaults PAGE(pg3,DSP__libBg){...} // Create Page with only image changed from defaults; DRAW(dr4,40,40,DSD_B__RED__BR); // Create Draw, type = box; only specifiying some parameters KEY(key2,func,100,100,DSK_C____TL); // Create Key, action = change; curRel = TL
|
|
49.51 |
Built-In TEXT, PAGE, DRAW, and IMAGE Styles |
10 Feb 14 |
|
A larger range of "default" styles has been added.
The built-in styles are prefixed by four characters as follows: * Text Styles are prefixed by "DST_" * Page Styles are prefixed by "DSP_" * Draw Styles are prefixed by "DSD_" * Image Styles are prefixed by "DSI_"
The styles are not created at start up. The style is only created the first time it is referenced from the users' project.
The style are created from the parameters within the style name itself, ie the colour black is taken from the actual name DSP_BLACK, so DSP_PINK would create a colour pink. These colours are from the colour table on the website.
Here are the rules/examples for each
Add a default TEXT style DST_ DST_col DST_col_fnt DST_col_fnt_len DST_col_fnt_len_row DST_col_fnt_len_row_cur Where col = 'col' name or 3-digit hex or 6-digit hex (see "Colours" below) fnt = 'font' 8, 16, 32 for Ascii8, Ascii16, Ascii32 or font name (see "Fonts" below) len = 'maxLen' row = 'maxRows' cur = 'curRel' Examples TEXT(txt1,"Hello",DST_BLACK_16); // Create Text, colour = black; font = built-in Ascii16; TEXT(txt2,"",DST_F30_fnt32_8_4); // Create Text, colour = \\FF3300, font = fnt32; maxLen = 8; maxRows = 4 TEXT(txt3,"",DST_RED_F32_8_4_TL); // Create Text, colour = \\FF3300, font = F32; maxLen = 12; maxRows = 4; curRel = TL
Add a default PAGE style DSP_ DSP_col DSP_col_img Where col = 'col' name or 3-digit hex or 6-digit hex (see "Colours" below) img = 'image' image name Examples PAGE(pg1,DSP_BLACK){…} // Create Page, back = black; PAGE(pg2,DSP_AB0056_libBg){…} // Create Page, back = \\AB0056, image = libBg;
Add a default DRAW style DSD_ DSD_typ DSD_typ_wid DSD_typ_wid_col DSD_typ_wid_col_fil DSD_typ_wid_col_fil_cur Where typ = 'type' of shape (best to use the single letter here) wid = 'width' of border col = 'col' colour of border (see "Colours" below) fil = 'back' colour of fill (see "Colours" below) cur = 'curRel' Examples DRAW(dr1,100,20,DSD_BOX); // Create Draw, type = box; DRAW(dr2,120,DSD_C_2_RED); // Create Draw, type = circle; width = 2; border colour = red; DRAW(dr3,60,40,DSD_B_1_RED_FD0_TL,20,40); // Create Draw, type = box; width = 1; border = red; back = \\FFDD00; curRel = TL;
Add a default IMAGE style DSI_ DSI_cur Where cur = 'curRel' Examples IMG(img1,libImg1,DSI_TL); // Create Image, curRel = TL;
The only limitations to this method is that the style name needs to fit within the 18 character entity name limit.
Colours Where the styles take a colour for a parameter, then the value can be represented in one of three ways. * The name of the colour can be specified as in the colour chart on the website, eg BLACK, BLUE, GOLD etc * A 6 digit hexadecimal number can be used, eg 123ABC which converts to \\123ABC * A 3 digit hexadecimal number can be used, eg FD0 which converts to \\FFDD00
Fonts Where the style takes a font for a parameter, then one of two options are assumed. * If the value is either 8, 16, or 32 then the built-in fonts Ascii8, Ascii16 or Ascii32 are used * Otherwise the value is assumed to be the name of a font loaded by the LIB command, eg fnt64 from LIB(fnt64,"SDHC/name.fnt");
Examples from testing:
PAGE(p1,DSP_){} STYLE(DSP_,PAGE){}
PAGE(p2,DSP_BLACK){} STYLE(DSP_BLACK,PAGE){back=BLACK;}
PAGE(p3,DSP_FD0){} STYLE(DSP_FD0,PAGE){back=\\FFDD00;}
PAGE(p4,DSP_ABC123){} STYLE(DSP_ABC123,PAGE){back=\\ABC123;}
LIB(Im1,"SDHC/page/pgMain_1.bmp"); PAGE(p5,DSP_000_Im1){} STYLE(DSP_000_Im1,PAGE){back=\\000000;image=Im1;}
PAGE(p6,DSP_blue_Im1){} STYLE(DSP_blue_Im1,PAGE){back=blue;image=Im1;}
IMG(i1,Im1,DSI_); STYLE(DSI_,IMAGE){}
TEXT(t1,"",DST_); STYLE(DST_,TEXT){}
TEXT(t2,"",DST_BLACK); STYLE(DST_BLACK,TEXT){col=BLACK;}
TEXT(t3,"",DST_blue_32); STYLE(DST_blue_32,TEXT){col=blue;font=Ascii32;}
LIB(Fnt16,"SDHC/asc_16b.fnt"); TEXT(t4,"Hello",DST_red_Fnt16); STYLE(DST_red_Fnt16,TEXT){col=red;font=Fnt16;}
TEXT(t5,"Hi",DST_fd0_Fnt16,4,5); STYLE(DST_fd0_Fnt16,TEXT){col=\\ffdd00;font=Fnt16;}
DRAW(d1,100,DSD_); STYLE(DSD_,DRAW){}
DRAW(d2,200,DSD_C); STYLE(DSD_C,DRAW){type=C;}
DRAW(d3,100,50,DSD_B); STYLE(DSD_B,DRAW){type=B;}
DRAW(d4,30,40,DSD_B_2); STYLE(DSD_B_2,DRAW){type=B;width=2;}
DRAW(d5,120,DSD_C_1_red); STYLE(DSD_C_1_red,DRAW){type=C;width=1;col=red;}
DRAW(d6,30,40,DSD_B_2_Red_Blue); STYLE(DSD_B_2_Red_Blue,DRAW){type=B;width=2;col=Red;back=Blue;}
DRAW(d7,10,30,0,140,DSD_E_20_FD0_FFF,30,30); STYLE(DSD_E_20_FD0_FFF,DRAW){type=E;width=20;col=\\FFDD00;back=\\FFFFFF;}
|
|
49.51 |
Problem with RESET( graph );; |
06 Feb 14 |
|
* Fixed problem where graph is not redrawn with RESET(graph);; when page update=changed. It was necessary to send SHOW(THIS_PAGE);
|
|
49.48 |
DRAW() with Structures |
29 Nov 13 |
|
* DRAW() command now accepts structure parameters for width/height/angles/offset.
|
|
49.48 |
Anti-Aliased Line Drawing |
29 Nov 13 |
|
* New algorithm for line drawing implemented. * Lines are created with anti-aliased edges. * Vertical and horizontal lines are a special case and are rapidly created (as no anti-aliasing required). * Line ends are now created perpendicular to the line rather than vertical or horizontal.
|
|
49.48 |
New Line Draw ''curRel'' Options Plus Offset |
29 Nov 13 |
|
* Additional parameter can now be passed to DRAW() command for Line and Vector drawing which allow for the line to be offset relative to the cursor: DRAW( name, length, angle, offset, stVect ); DRAW( name, length, angle, offset ); DRAW( name, length, angle, offset, stVect, xPos, yPos ); DRAW( name, width, height, offset, stLine ); DRAW( name, width, height, offset ); DRAW( name, width, height, offset, stLine, xPos, yPos ); * The offset parameter is ideal for the pointer on gauges and clocks where the point of rotation is a certain distance along the line * New curRel options allow for better line placement. SA, SB, SC // Start of line. SA = Edge A; SB = Edge B; SC = Centre EA, EB, EC // End of line. EA = Edge A; EB = Edge B; EC = Centre MA, MB, MC // Mid-point of line. MA = Edge A; MB = Edge B; MC = Centre OA, OB, OC // Offset along line. OA = Edge A; OB = Edge Bl OC = Centre
* Example: Vector length = 100, angle = 125 deg, width = 5 (not to scale)
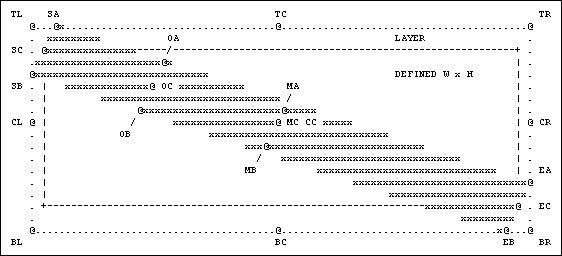
|
|
49.48 |
Ellipses Support Degrees & Radians |
11 Nov 13 |
|
* Added option for start angle and arc angle to be in radians or degrees depending on SYSTEM.angles setting.
|
|
49.48 |
Drawing Vectors (Lines with Length and Angle) |
11 Nov 13 |
|
* Added new line type - vector - which is defined with a length and an angle. STYLE( stVector, DRAW ) { type = v or vector; ... } DRAW( name, length, angle, stVector );
where 'length' is line length, 'angle' is angle clockwise from 12'o'clock position. 'angle' is in degrees or radians depending on SYSTEM.angles setting.
Example tu800a.mnu
STYLE(stPage,PAGE){} STYLE(stLine1,DRAW){type=vector;col=white;width=1;curRel=cc;} STYLE(stLine2,DRAW){type=vector;col=white;width=2;curRel=cc;} STYLE(stLine3,DRAW){type=vector;col=white;width=3;curRel=cc;} STYLE(stLine4,DRAW){type=v;col=white;width=9;curRel=cc;} STYLE(stLine5,DRAW){type=v;col=white;width=20;curRel=cc;} STYLE(stLine6,DRAW){type=v;col=white;width=21;curRel=cc;} STYLE(stCirc,DRAW){type=circle;width=1;col=red;curRel=cc;}
VAR(varAngle,0.0,FLT4);
PAGE(pgMain,stPage) { POSN(149,119); DRAW(drLine1,200,45,stLine1); DRAW(drCir1,150,stCirc); DRAW(drPt1,7,stCirc); POSN(399,119); DRAW(drLine2,200,45,stLine2); DRAW(drCir2,150,stCirc); DRAW(drPt2,7,stCirc); POSN(649,119); DRAW(drLine3,200,45,stLine3); DRAW(drCir3,150,stCirc); DRAW(drPt3,7,stCirc); POSN(149,359); DRAW(drLine4,200,45,stLine4); DRAW(drCir4,150,stCirc); DRAW(drPt4,7,stCirc); POSN(399,359); DRAW(drLine5,200,45,stLine5); DRAW(drCir5,150,stCirc); DRAW(drPt5,7,stCirc); POSN(649,359); DRAW(drLine6,200,45,stLine6); DRAW(drCir6,150,stCirc); DRAW(drPt6,7,stCirc); LOOP(lpMain,FOREVER) { DRAW(drLine1,150,varAngle); DRAW(drLine2,150,varAngle); DRAW(drLine3,150,varAngle); DRAW(drLine4,150,varAngle); DRAW(drLine5,150,varAngle); DRAW(drLine6,150,varAngle);; CALC(varAngle,varAngle,0.5,"+"); } } SHOW(pgMain);
|
|
49.46 |
Style switching LOAD(text.style,newstyle); |
21 Oct 13 |
|
* Added functionality to allow the style of an entity to be changed to another. LOAD(entName.style,newStyle);
eg STYLE(stText1,TEXT){ font=Ascii16; col=blue; back=dimgrey; } STYLE(stText2,TEXT){ font=Ascii8; col=green; back=mistyrose; } ... TEXT(txtTest,"This is a test",stText1); ... LOAD(txtTest.style,stText2);;
* Works with STYLES: TEXT, DRAW, IMAGE, PAGE, KEY.
Test Example:
SETUP(RS2){set="1152ND";encode=sr;}
LIB(libImg1,"SDHC/sunflower120x122-4.png");
STYLE(stPage1,PAGE){} STYLE(stPage2,PAGE){ back=pink; } STYLE(stText1,TEXT){ font=Ascii16; col=blue; back=dimgrey; maxLen=128; padding=8; width=4; bcol=aqua; } STYLE(stText2,TEXT){ font=Ascii8; col=green; back=mistyrose; maxLen=128; padding=2; width=1; bcol=red; } STYLE(stDraw1,DRAW){ type=box; col=aqua; back=dimgrey; width=4; maxX=200; maxY=200; } STYLE(stDraw2,DRAW){ type=circle; col=red; back=mistyrose; width=1; maxX=200; maxY=200; } STYLE(stImg1,IMAGE){ curRel=TL; rotate=0; } STYLE(stImg2,IMAGE){ curRel=CC; rotate=180; } STYLE(stTouch1,KEY){type=touch; action=c; repeat=100; evfunc=fnKey1; } STYLE(stTouch2,KEY){type=touch; action=c; repeat=1000; evfunc=fnKey2; }
STYLE(stBtnTxt,TEXT){ font=Ascii16; col=white; } STYLE(stBtnBox,DRAW){ type=box; col=silver; back=grey; width=2; }
VAR(vSw,0,U8);
PAGE(pgMain,stPage1) { POSN(59, 25); DRAW(drwBtn1,100,30,stBtnBox); TEXT(txtBtn1,"Next Style",stBtnTxt); KEY(keyBtn1,[IF(vSw==0?fnSw0:fnSw1);;],100,30,TOUCH);
POSN(109,175); TEXT(txtSam1,"ABCDEFGHIJ",stText1); POSN(109,215); TEXT(txtSam2,"ABCDEFGHIJ",stText2);
POSN(239,55); DRAW(drwShp1,100,80,stDraw1); POSN(239,155); DRAW(drwShp2,100,80,stDraw2);
POSN(369,99); IMG(imgFlw1,libImg1,stImg1); POSN(369,171); IMG(imgFlw2,libImg1,stImg2);
POSN(59,125); DRAW(drwBtn2,100,30,stBtnBox); TEXT(txtBtn2,"Test",stBtnTxt); KEY(keyBtn2,[LOAD(RS2,"D");],[LOAD(RS2,"U");],[LOAD(RS2,"R");],100,30,stTouch1);
} SHOW(pgMain);
FUNC(fnSw0) { LOAD(pgMain.style,stPage2); LOAD(txtSam1.style,stText2); LOAD(txtSam2.style,stText1); LOAD(drwShp1.style,stDraw2); LOAD(drwShp2.style,stDraw1); LOAD(imgFlw1.style,stImg2); LOAD(imgFlw2.style,stImg1); LOAD(keyBtn2.style,stTouch2); LOAD(vSw,1); }
FUNC(fnSw1) { LOAD(pgMain.style,stPage1); LOAD(txtSam1.style,stText1); LOAD(txtSam2.style,stText2); LOAD(drwShp1.style,stDraw1); LOAD(drwShp2.style,stDraw2); LOAD(imgFlw1.style,stImg1); LOAD(imgFlw2.style,stImg2); LOAD(keyBtn2.style,stTouch1); LOAD(vSw,0); }
FUNC(fnKey1) { LOAD(RS2,"1"); }
FUNC(fnKey2) { LOAD(RS2,"2"); }
VAR(vCal,0,U8); LOAD(vCal,TCH_CAL); IF( vCal==0?[LOAD(SYSTEM.calibrate,y);]);
|
|
49.42 |
Filling Ellipses and Arcs Overflowing |
06 Sep 13 |
|
* Addressed issues found with ellipses with small borders incorrectly being filled * Fixed problem with fill overflowing with small angles. Angles of less than 2 degrees now rendered as a number of radial lines rather than using the fill algorithm.
|
|
49.42 |
Graphs to support alpha blending and opacity |
06 Sep 13 |
|
* New features of alpha blending and opacity with graphs * Support for graphs when the display is rotated 90, 180 and 270 degrees
|
|
49.42 |
DRAWing of Graphs - Trace Line Should Not be Wrapping |
06 Sep 13 |
|
* Improved graph trace drawing. * Solved problem with trace line wrapping when rendering a graph. * Extended graphics area to encompass line width and modified page rendering to deal with windowed pixels.
|
|
49.37 |
DRAWing of Ellipses and more DRAW parameter options |
10 Jun 13 |
|
* Added ellipse drawing, specify type=ellipse or e in the STYLE * Added more DRAW command options DRAW( name, x ); // Circle or Square modification: width=x; height=x; DRAW( name, x, style ); // Circle or Square definition: width=x; height=x; DRAW( name, x, y ); // All Shapes modification: width=x; height=y; DRAW( name, x, y, style ); // All Shapes definition: width=x; height=y; DRAW( name, x, y, s, a ); // Ellipse Arc modification: width=x; height=y; startAngle=s; arcAngle=a; DRAW( name, x, y, s, a, style ); // Ellipse Arc definition: width=x; height=y; startAngle=s; arcAngle=a; * Notes: - 0 degrees is at 12'o'clock and positive angles are clockwise. - The style for an arc must be defined to encompass the size of the shape if drawn from 0 to 360 degrees. - Further work required on the fill algorithm for ellipses.
|
|
49.37 |
Colours of ''none'' or ''transparent'' |
10 Jun 13 |
|
* The value of "none" or "transparent" can now be used for all colours in the styles.
|
|
49.37 |
Graph Displaying Problems |
10 Jun 13 |
|
* Added bounds check to prevent drawing outside of graph area. * Fixed line wrapping within graph area.
|
|
49.37 |
POSN in DRAW, IMG, TEXT and KEY Commands |
10 Jun 13 |
|
* Added initial positioning to commands: a) DRAW(name,width,style,x,y); DRAW(name,width,height,style,x,y); DRAW(name,width,height,start,arc,style,x,y); b) IMG(name,src,style,x,y); c) TEXT(name,text,style,x,y); d) KEY(name,func,width,height,style,x,y); KEY(name,downFunc,upFunc,width,height,style,x,y); KEY(name,downFunc,upFunc,repFunc,width,height,style,x,y);
|
|
49.25 |
Draw Line - Drawing of vertical and horizontal lines fixed. |
01 Nov 12 |
|
Draw Line * Drawing of vertical and horizontal lines fixed.
|
|
49.19 |
DRAW() Height/Width of Zero - Lines/circles/boxes no longer rendered if height or width equals zero. |
05 Oct 12 |
|
Lines/circles/boxes no longer rendered if height or width equals zero.
|
|
49.14 |
Opacity - Corrected problem with assigning initial opacity value to text, images, draw, pages. |
27 Jul 12 |
|
* Corrected problem with assigning initial opacity value to text, images, draw, pages.
|
|
49.00 |
Opacity - Added opacity to images, drawing objects and text. |
22 Mar 12 |
|
Added opacity to images, drawing objects and text STYLE( st, image | draw | text ) { ...... opacity = n; // n = 0..100 where 0=transparent..100=opaque (default=100) ....... }
LOAD( st.opacity, num ); // Dot operator also supported. Restrictions: Not supported for library images with bits=16.
|
|
48.24 |
DRAW() - Fixed problem where width/height of shape is specified as 0. |
10 Mar 12 |
|
Fixed problem where width/height of shape is specified as 0. Fix to report error if style name not valid in DRAW() command.
|
|
47.24 |
Text, Draw and Image Entity Information |
31 Oct 11 |
|
More Calc commands added to obtain entity information > CALC(var,ename,"ESIZE"); -> returns allocated display size in bytes > CALC(var,ename,"EDEL"); -> returns 1 if entity has been deleted, else 0 > CALC(var,ename,"EVIS"); -> returns 1 if entity is visible, else 0 > CALC(var,ename,"EALIGN"); -> returns value representing alignment: 0 = Top Left, 1 = Top Centre, 2 = Top Right, 3 = Centre Left, 4 = Centre Centre, 5 = Centre Right, 6 = Bottom Left, 7 = Bottom Centre, 8 = Bottom Right
|
|
47.00 |
Graphs - A number of graph styles now exist as draw types. |
09 Jul 11 |
|
A number of graph styles now exist as draw types: type=p; type=pixel; // Pixel Scatter - places a point at x,y type=t; type=trace; // Trace/Line - joins the dots between current point and previous point. type=y; type=yBar; // Bar Y - draws vertical line from 0 to y and clears from y+1 to ymax type=x; type=xBar; // Bar X - draws horizontal line from 0 to x and clears from x+1 to xmax The origin on the graph can be changed xOrigin=val; // (default=0) yOrigin=val; // (default=0)
The scaling of pixels can be set: xScale=val; // (default=100.0) [val can be float and is a percentage] yScale=val; // (default=100.0) [val can be float and is a percentage] Note to draw graph with 0,0 at top and n,n at bottom, use yScale=-100;
The graph can be made to scroll (currently right-to-left only supported) xScroll=val; // where val=0 (default - no scroll); val=n (scroll left n pixels before each plot
STYLE(gstyle,DRAW) { type=trace; maxX=100; maxY=100; col=green; back=black; width=3; curRel=cc; xOrigin=50; yOrigin=50; xScale=200; yScale=200; xScroll=1; }
|
|
42.00 |
Draw Graph - Graph drawing added. |
09 Mar 11 |
|
Graph drawing added, creating dot colour, background colour, max width, max height and dot size using draw style draw(name,sizeX,sizeY,style); creates graph area draw(name,posX,posY); puts dot in graph area at posX, posY (0,0 is top left reset(name); clears graph To be done - graph scrolling / redrawing, dot overlay / replace column
|
|
38.00 |
Draw - Box rotation of 0, 90, 180 and 270 degrees now supported. |
13 Jan 11 |
|
Box rotation of 0, 90, 180 and 270 degrees now supported. Example: STYLE( boxStyle, draw ) { rotate=180; } Line drawing added. Example: DRAW( line1, 10, 10, lineStyle ); Draws line 45 degrees top left to bottom right Example: DRAW( line2, 10, -10, lineStyle ); Draws line 45 degrees bottom left to top right Line rotation 0, 90, 180 and 270 degrees added. · Pixel drawing added.
|
|
36.00 |
Drawing - Fixed problem with multiple instances of same shape being created when a shape is resized eg DRAW(box,10,10). |
23 Nov 10 |
|
Fixed problem with multiple instances of same shape being created when a shape is resized eg DRAW(box,10,10). Corrected allocation of memory for drawing objects (using maxX and maxY) Returns error if shape size is increased beyond size allocated.
|
|
35.00 |
DRAW - DRAW now accepts VARs, signed/unsigned integers and pointers. |
12 Nov 10 |
|
DRAW now accepts VARs, signed/unsigned integers (U8, U16, U32, S8, S16, S32), floats (FLT) and pointers (PTR) DRAW( PTR, VAR|INT|FLT|PTR, VAR|INT|FLT|PTR, Style ); Note PTR refers to the entity being pointed to by PTR and not the PTR itself. Use LOAD( PTR > "Name" ); to set a pointer.
|
|
32.00 |
VAR - VAR(Name,Style); changes to VAR(Name, default Value, Style);. |
14 Oct 10 |
|
Due to customer request, including the default value reduces the lines of code and matches other command types like TEXT, IMG, DRAW. VAR(Name,Style); changes to VAR(Name, default Value, Style); Example1: VAR( MyVarU8, 0, U8 ); Example2: VAR( MyVarTxt, "Hello", TXT ); Example3: VAR( MyVarPtr > "ImgABC", PTR ); A new built in style TXT can be used for text variables with default 32 characters length
|
|
21.00 |
STYLE - Fixed error with default style initialization. |
30 Jul 10 |
|
Fixed error with default style initialization. Style parameter names for POSN, PAGE, TEXT, DRAW, IMAGE, KEY set up
|
|
19.00 |
DRAW - Fixed border and fill colour displaying for shapes and drawing of circle with ‘odd’ sized diameter |
14 Jul 10 |
|
Fixed border and fill colour displaying for shapes Fixed drawing of circle with ‘odd’ sized diameter
|
|
|
|